PYTHON PROGRAM THAT ASKS USER FOR LARGE INTEGERS AND INSERTS COMMAS INTO IT ACCORDING TO THE STANDARD AMERICAN CONVENTION FOR COMMAS IN LARGE NUMBERS
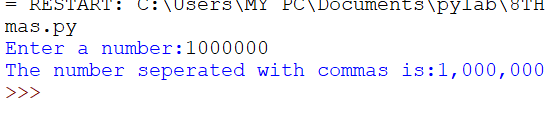
Write a program that asks the user for a large integer and inserts commas into it according to the standard American convention for commas in large numbers. For instance, if the user enters 1000000, the output should be 1,000,000 CODE: n=int(input("Enter a number:")) print("The number seperated with commas is:{:,}".format(n)) EXPLANATION: The format() method formats the specified value(s) and insert them inside the string's placeholder. The placeholder is defined using curly brackets: {} Inside the placeholders you can add a formatting type to format the result SYNTAX: string .format( value1, value2... ) PARAMETER VALUES: Parameter Description value1, value2... Required. One or more values that should be formatted and inserted in the string. The values can be A number specifying the position of the element you want to remove. The values are either a list of values separated by commas, a key=value list, or a combination of both. The values can be of any data ...